Laravel Pipeline: A Powerful Tool for Managing Data Flows
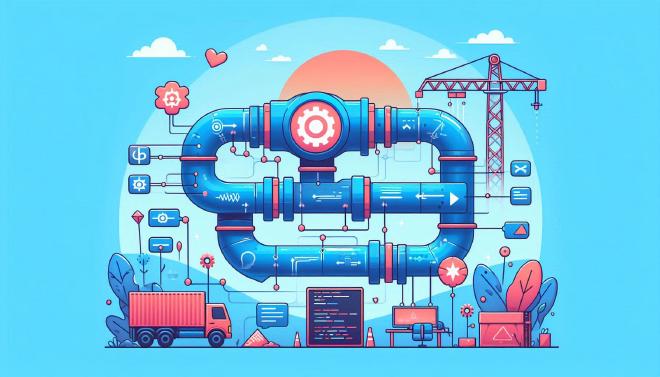
Table of Contents
What is Laravel Pipeline and How Does It Work? #
Pipeline is an implementation of the Pipeline Pattern, which allows an object to pass through a series of handlers, each performing a specific task. This is a great way to structure code following the Single Responsibility Principle (SRP), which is part of SOLID.
Key Features of Pipeline #
Pipeline is especially useful in scenarios like:
- Object transformation – when you need to apply multiple handlers to modify or enrich an object.
- Model processing before saving or after updating – when you need to execute several independent processes, such as creating relationships, sending notifications, or integrating with third-party services.
Example of Laravel Pipeline Usage #
Let’s say that after creating a user, we need to:
- Generate a profile picture;
- Activate a subscription;
- Send a welcome email.
Instead of handling this logic in one place, Pipeline can be used:
use Illuminate\Pipeline\Pipeline;
$user = Pipeline::send($user)
->through([
GenerateProfilePhoto::class,
ActivateSubscription::class,
SendWelcomeEmail::class,
])
->then(fn (User $user) => $user);
Each handler is a separate class, like this:
class GenerateProfilePhoto {
public function handle(User $user, Closure $next) {
// Generate profile photo
$user->profile_photo = 'default.png';
return $next($user);
}
}
The key takeaway: each class receives the $user
object, performs its logic, and passes it to the next handler using $next($user)
. This is similar to Middleware but can be used beyond HTTP requests, in any business logic.
Why Use Pipeline? #
- Cleaner and more readable code – each process is in its own class.
- Flexibility – easily add or remove handlers.
- Reusability – the same classes can be used in different parts of the application.
Additional Resources #
🔗 Laravel Pipeline Documentation
Conclusion #
If you need to process data sequentially, Pipeline is a great way to do it efficiently and elegantly. Now that this tool is officially documented in Laravel 10, using it is easier than ever.